GraphQL
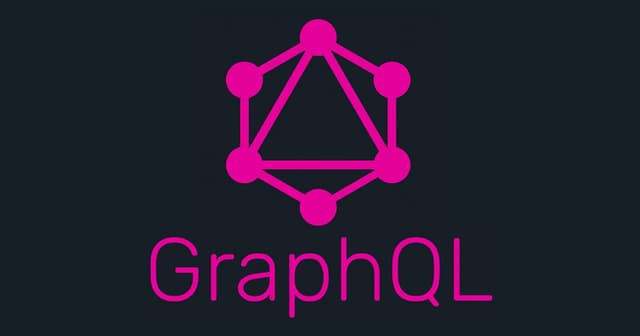
Understanding GraphQL: A Comprehensive Guide
GraphQL has emerged as a powerful alternative to traditional REST APIs, offering developers a flexible and efficient way to interact with data. With its precise data fetching capabilities, single endpoint architecture, and strongly typed schema, GraphQL is becoming increasingly popular in modern web and mobile applications. In this blog post, we will explore the key features of GraphQL, how it compares to REST, and provide practical examples to help you get started.
Key Features of GraphQL
1. Precise Data Fetching
One of the standout features of GraphQL is its ability to let clients specify exactly what data they need. This reduces the common problems of over-fetching and under-fetching.
- Over-fetching occurs when an API returns more data than necessary, consuming bandwidth and processing time. For instance, requesting user data might return additional information like addresses or phone numbers that the client does not need.
- Under-fetching happens when a single request does not return enough data, forcing clients to make multiple requests. For example, if you only receive user data without their recent posts, you’ll need to fetch that data separately.
2. Single Endpoint
Unlike REST APIs, which typically require multiple endpoints for different resources, GraphQL operates through a single endpoint. This simplifies the architecture and reduces the complexity of managing multiple routes.
3. Strongly Typed Schema
GraphQL APIs are defined by a schema that clearly specifies the types of data and their relationships. This schema acts as a contract between the client and server, ensuring that queries are valid and predictable. Here’s a simple example:
type Author { id: ID! name: String! books: [Book!]! } type Book { id: ID! title: String! author: Author! } type Query { authors: [Author!]! books: [Book!]! book(id: ID!): Book author(id: ID!): Author }
In this schema:
- Author and Book are object types with fields.
- The Query type defines how clients can access data.
4. Real-time Data
GraphQL supports subscriptions, allowing clients to receive real-time updates. This is particularly useful for applications that require live data, such as chat applications or collaborative platforms.
5. Tooling and Ecosystem
The GraphQL ecosystem includes various tools that enhance development. GraphiQL, for example, is an in-browser IDE that allows developers to explore GraphQL APIs, write queries, and visualize data.
How GraphQL Works
Defining Your Data (Schema)
In GraphQL, you begin by defining a schema that outlines the types of data available. This schema serves as the foundation for your API.
Writing Queries
Once the schema is established, clients can write queries to request specific data. For instance, to fetch a list of authors and their books:
{ authors { name books { title } } }
Receiving Predictable Results
The server responds with data that matches the structure of the query, making it easy to anticipate the response format. For example:
{ "data": { "authors": [ { "name": "Author 1", "books": [{ "title": "Book 1" }, { "title": "Book 2" }] }, { "name": "Author 2", "books": [{ "title": "Book 3" }] } ] } }
GraphQL vs. REST
Performance
GraphQL can be more efficient due to its precise data fetching capabilities. However, complex queries may lead to performance bottlenecks on the server. REST, while generally simpler, may not always optimize data transfer as effectively.
Flexibility
GraphQL’s flexibility allows clients to evolve independently of the server, making it easier to add new features without breaking existing functionality. REST APIs may require client updates for changes, limiting flexibility.
Tooling
Both GraphQL and REST have robust ecosystems. GraphQL benefits from tools like Apollo and Relay, while REST has a mature set of libraries and tools across various programming languages.
Use Cases
- GraphQL is ideal for applications needing complex data fetching, real-time updates, or rapid feature evolution.
- REST is suitable for simpler applications or microservices where caching and performance are paramount.
Building a GraphQL Server with Node.js
To set up a GraphQL server in Node.js, you can use the
apollo-server-express
package. Here’s a quick example:
const { ApolloServer, gql } = require("apollo-server-express"); const express = require("express"); const typeDefs = gql` type Query { hello: String } `; const resolvers = { Query: { hello: () => "Hello world!", }, }; const server = new ApolloServer({ typeDefs, resolvers }); const app = express(); server.applyMiddleware({ app }); app.listen({ port: 4000 }, () => console.log(`Server ready at http://localhost:4000${server.graphqlPath}`) );
Using GraphiQL
GraphiQL is an excellent tool for exploring your GraphQL API. It allows you to write and test queries in an interactive environment.
Designing a Schema for E-commerce
For an e-commerce application, your GraphQL schema might look like this:
type Query { products: [Product] product(id: ID!): Product } type Product { id: ID! name: String! price: Float! reviews: [Review] } type Review { id: ID! content: String! rating: Int! }
Conclusion
GraphQL presents a powerful approach to API design, addressing common challenges faced by developers using REST. Its flexibility, precise data fetching, and real-time capabilities make it an excellent choice for modern applications. Whether you’re building a new project or considering a transition from REST, understanding GraphQL will undoubtedly enhance your development toolkit.
Additional Resources
- Apollo Documentation: Explore Apollo's comprehensive guides and tutorials.
- GraphQL Specification: Dive deeper into the technical specifications of GraphQL.
- GraphiQL: Use this tool to test and explore GraphQL APIs interactively.
By leveraging GraphQL, you can create more efficient, maintainable, and user-friendly applications. Happy coding!