Performance improvement in Nodejs
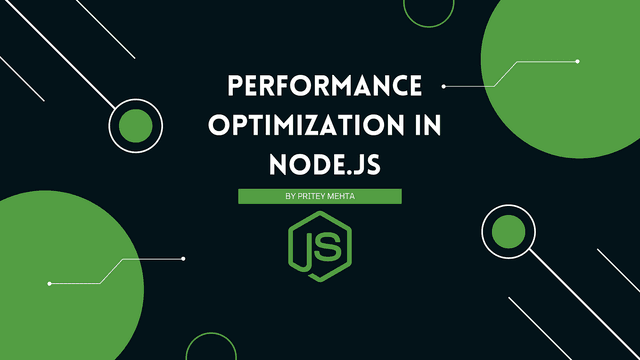
Comprehensive Guide to Enhancing Node.js Performance
Node.js is a powerful platform for building scalable network applications. However, to truly harness its potential, you must optimize its performance. This guide delves into various strategies and best practices to enhance Node.js performance, covering everything from asynchronous programming to advanced process management.
1. Asynchronous Programming
Node.js's non-blocking, event-driven architecture allows it to handle many operations concurrently. To fully leverage this feature, you must use asynchronous programming techniques effectively.
Using async/await and Promises
-
Promises: Promises represent the eventual completion (or failure) of an asynchronous operation. They help manage asynchronous tasks and avoid "callback hell".
const fs = require("fs").promises; async function readFile() { try { const data = await fs.readFile("file.txt", "utf8"); console.log(data); } catch (error) { console.error(error); } } readFile();
-
async/await: async functions always return a Promise. await is used to pause the execution until the Promise is resolved.
async function fetchData() { let response = await fetch("https://api.example.com/data"); let data = await response.json(); console.log(data); } fetchData();
Benefits
- Avoids blocking the event loop.
- Makes asynchronous code easier to read and maintain.
2. Event Loop Monitoring
The event loop is central to Node.js’s performance. If it’s blocked, your application will become unresponsive.
Monitoring Tools
- Node.js Profiler: Use node --inspect or node --inspect-brk to start the Node.js Inspector.
- clinic: Tools like Clinic.js help diagnose performance issues.
- Performance Hooks: Node.js provides built-in performance hooks for monitoring.
Example
To check the status of the event loop, you can use the node-event-loop-monitor package:
npm install node-event-loop-monitor
const monitor = require("node-event-loop-monitor"); monitor.start(); setInterval(() => { console.log(monitor.getStats()); }, 1000);
3. Memory Management
Effective memory management helps avoid leaks and optimize usage.
Detecting Memory Leaks
- heapdump: Capture and analyze heap snapshots.
npm install heapdump
const heapdump = require("heapdump"); heapdump.writeSnapshot("./myapp-" + Date.now() + ".heapsnapshot");
- node-inspect: Use Node’s built-in inspector for real-time memory profiling.
Optimizing Memory Usage
- Avoid global variables that can lead to leaks.
- Use proper data structures and avoid unnecessary memory consumption.
4. Non-blocking I/O
Non-blocking I/O operations prevent the event loop from being blocked.
Asynchronous Methods
- File System Operations: Use fs.promises or fs.createReadStream for non-blocking file I/O.
const fs = require("fs").promises; async function readFile() { try { const data = await fs.readFile("largefile.txt"); console.log(data.toString()); } catch (error) { console.error(error); } } readFile();
- Database Queries: Ensure database operations are non-blocking by using asynchronous drivers.
Advantages
- Improved responsiveness and scalability.
- Better utilization of system resources.
5. Efficient Data Handling
Managing large datasets efficiently is crucial for performance.
Using Streams
- File Streams: Handle large files with streams to avoid high memory consumption.
const fs = require("fs"); const readStream = fs.createReadStream("largefile.txt"); readStream.on("data", (chunk) => { console.log("Received chunk:", chunk); }); readStream.on("end", () => { console.log("File read complete"); });
- HTTP Streams: Stream responses to clients for better performance.
Benefits
- Reduces memory footprint.
- Allows processing of data in chunks, improving performance.
6. Caching
Caching reduces the need for repeated computations and database queries.
Types of Caching
- In-memory Cache: Use libraries like node-cache for storing frequently accessed data.
npm install node-cache
const NodeCache = require("node-cache"); const cache = new NodeCache(); cache.set("key", "value"); console.log(cache.get("key"));
- External Cache: Use Redis for distributed caching.
npm install redis
const redis = require("redis"); const client = redis.createClient(); client.set("key", "value", redis.print); client.get("key", (err, reply) => { console.log(reply); });
Advantages
- Reduces latency by avoiding repetitive operations.
- Enhances overall application performance.
7. Load Balancing
Distributing incoming network traffic across multiple servers or processes helps prevent bottlenecks.
Methods
- Hardware Load Balancers: Use physical devices to distribute traffic.
- Software Load Balancers: Tools like Nginx or HAProxy provide efficient load balancing solutions.
- Built-in Node.js Features: Utilize the cluster module for process-level load balancing.
Example with Nginx
Configure Nginx as a reverse proxy to balance load between multiple Node.js instances:
http { upstream node_app { server 127.0.0.1:3000; server 127.0.0.1:3001; } server { listen 80; location / { proxy_pass http://node_app; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; proxy_set_header X-Forwarded-Proto $scheme; } } }
Benefits
- Prevents any single server from becoming a performance bottleneck.
- Enhances application availability and reliability.
8. Clustering
The cluster module in Node.js allows you to create multiple processes to handle incoming requests concurrently.
Basic Example
const cluster = require("cluster"); const http = require("http"); const numCPUs = require("os").cpus().length; if (cluster.isMaster) { for (let i = 0; i < numCPUs; i++) { cluster.fork(); } cluster.on("exit", (worker, code, signal) => { console.log(`Worker ${worker.process.pid} died`); }); } else { http .createServer((req, res) => { res.writeHead(200); res.end("Hello, World!"); }) .listen(8000); }
Advantages
- Utilizes multiple CPU cores to improve performance.
- Enhances fault tolerance by distributing load across worker processes.
9. Reverse Proxy
A reverse proxy handles incoming requests and forwards them to backend servers, providing benefits such as SSL termination and load balancing.
Benefits
- Offloads SSL/TLS encryption from backend servers.
- Distributes traffic to prevent overload on any single server.
Popular Solutions
- Nginx: Widely used as a reverse proxy and load balancer.
- HAProxy: Known for its high performance and configurability.
10. HTTP/2 and SSL/TLS
HTTP/2 improves performance with features like multiplexing and header compression, while SSL/TLS ensures secure communication.
Enabling HTTP/2
In Nginx, enable HTTP/2 by adding the http2 parameter:
server { listen 443 ssl http2; ssl_certificate /path/to/cert.pem; ssl_certificate_key /path/to/key.pem; }
Benefits
- Reduced latency with multiplexing.
- Improved security with encryption.
11. WebSockets
WebSockets provide a persistent connection between the server and client, allowing real-time communication.
Basic WebSocket Server
const WebSocket = require("ws"); const wss = new WebSocket.Server({ port: 8080 }); wss.on("connection", (ws) => { ws.on("message", (message) => { console.log(`Received message => ${message}`); }); ws.send("Hello, Client!"); });
Advantages
- Real-time data transfer with low latency.
- Efficient for applications requiring frequent updates, such as chat applications or live notifications.
12. Memory Management
Effective memory management helps prevent leaks and optimize performance.
Tools
- heapdump: Capture heap snapshots to analyze memory usage.
- node-inspect: Monitor memory in real-time.
Best Practices
- Use local variables and avoid global state.
- Regularly profile and optimize memory usage.
13. CPU Usage
Offloading CPU-intensive tasks to worker threads or separate processes can improve performance.
Using Worker Threads
const { Worker } = require("worker_threads"); const worker = new Worker("./worker.js"); worker.on("message", (message) => { console.log(message); }); worker.postMessage("Start computation");
Worker Thread Example
// worker.js const { parentPort } = require("worker_threads"); parentPort.on("message", (message) => { let result = 0; for (let i = 0; i < 1e9; i++) { result += i; } parentPort.postMessage(result); });
Advantages
- Keeps the main event loop responsive.
- Utilizes multiple CPU cores effectively.
14. PM2 Process Manager
PM2 is a robust process manager for Node.js that simplifies monitoring, clustering, and deployments.
Installation
npm install pm2 -g
Basic Commands
- Start Application with Clustering:
pm2 start app.js -i max
- Monitor Processes:
pm2 monit
- Zero Downtime Restart:
pm2 reload all
Benefits
- Simplifies process management and clustering.
- Provides monitoring and zero-downtime deployment features.
Conclusion
Optimizing Node.js performance involves a combination of efficient coding practices, leveraging built-in features like clustering and worker threads, and using advanced tools like PM2 for process management. By implementing these strategies, you can build high-performance, scalable Node.js applications that can handle increased loads and provide a better user experience.