Security and Authentication in Nodejs
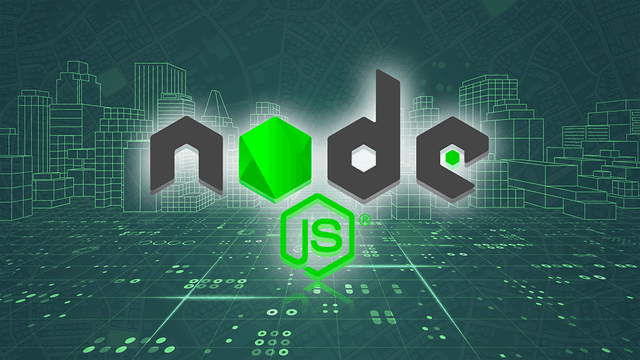
Securing Your Node.js Application: Best Practices for Authentication, Authorization, and OAuth Implementation
In today’s digital landscape, securing your Node.js application is paramount for protecting user data and maintaining application integrity. This guide provides a comprehensive overview of authentication, authorization, and the implementation of OAuth, detailing best practices, key concepts, and practical implementations.
Understanding Authentication and Authorization
Before diving into implementation, it’s crucial to distinguish between authentication and authorization:
- Authentication: This process verifies the identity of a user or system.
- Authorization: This determines what an authenticated user is allowed to do.
Key Questions to Consider
- Authentication: “Who are you?”
- Authorization: “What are you allowed to do?”
Example Scenario
- Authentication: A person uses a key card to enter a secure building.
- Authorization: Based on their role, the person can access specific areas (e.g., office access vs. server room access).
Implementing Authentication in Node.js
User Registration
To implement authentication, start with user registration:
- Create a Registration Form: Collect user data.
- Password Hashing: Use libraries like
to hash and salt passwords before storing them in the database.bcrypt
- Secure Storage: Store user data securely in a database.
User Login
Next, implement user login functionality:
- Login Form: Allow users to input credentials.
- Credential Validation: Validate credentials against stored data.
- Session Creation: If valid, create a session or generate a JSON Web Token (JWT) to maintain user login status.
Password Reset
Implement a secure password reset feature that involves email verification to enhance user security.
Implementing Authorization
Role-Based Access Control (RBAC)
RBAC allows you to define user roles (e.g., Admin, Editor, User) and assign specific permissions to each role. Use middleware to verify user roles and permissions before granting access to certain routes.
Protecting Routes
Secure specific routes by employing middleware functions that check user roles and permissions, ensuring that unauthorized users cannot access restricted resources.
Libraries for Authorization
Utilize libraries like
express-jwt
or passport
for token-based authentication and authorization.
Best Practices for Security
Password Hashing
Always hash and salt passwords to protect user credentials from data breaches. This is a fundamental practice for maintaining user security.
Use HTTPS
Secure data transmission using HTTPS to prevent eavesdropping and man-in-the-middle attacks.
Session Management
Implement secure session management practices to protect against session hijacking.
OAuth and Social Logins
Allow users to log in using OAuth providers (e.g., Google, Facebook), which not only enhances security but also improves user experience.
Vigilance with Third-Party Libraries
Be cautious when using third-party libraries to avoid introducing vulnerabilities into your application.
Denial of Service (DoS) Protection
Utilize a reverse proxy to handle incoming requests and configure server timeouts to mitigate potential DoS attacks.
Understanding SSL/TLS
Overview
SSL (Secure Sockets Layer) and TLS (Transport Layer Security) are protocols for establishing encrypted connections between clients and servers, ensuring secure communication.
Handshake Process
The SSL/TLS handshake process consists of the following steps:
- Client requests a secure connection.
- Server responds with its SSL/TLS certificate.
- Client verifies the certificate with a trusted Certificate Authority (CA).
- Client generates a session key, encrypts it with the server’s public key, and sends it to the server.
- Both parties use the session key for subsequent encrypted communication.
Symmetric vs. Asymmetric Encryption
Symmetric Encryption
- Key Usage: One key for both encryption and decryption.
- Speed: Generally faster due to simpler algorithms.
- Security: Requires secure key management to maintain confidentiality.
Asymmetric Encryption
- Key Usage: A pair of keys (public and private) is utilized.
- Speed: Slower due to more complex algorithms.
- Security: Simplifies key distribution and enhances security for data transmission.
Implementing SSL/TLS in Node.js
Obtaining an SSL/TLS Certificate
Acquire a certificate from a trusted CA (e.g., Let’s Encrypt) to ensure secure communication.
Setting Up HTTPS Server
Use the
https
module in Node.js to set up a secure server:
const https = require("https"); const fs = require("fs"); const express = require("express"); const app = express(); const options = { key: fs.readFileSync("path/to/private-key.pem"), cert: fs.readFileSync("path/to/certificate.pem"), }; https.createServer(options, app).listen(443, () => { console.log("HTTPS server running on port 443"); }); app.get("/", (req, res) => { res.send("Hello, secure world!"); });
Best Practices for SSL/TLS
- Store certificates securely and restrict access to prevent unauthorized use.
- Regularly update certificates to avoid expiration.
- Use strong cipher suites and enable HTTP Strict Transport Security (HSTS) for enhanced security.
The Role of Digital Certificates
Digital Certificates
Digital certificates verify the ownership of a public key and include relevant information about the key and the entity to which it belongs.
Certificate Signing
Certificates are issued by trusted CAs and signed with their private key, ensuring authenticity and integrity.
Preventing MITM Attacks
Clients verify server certificates to prevent unauthorized access and maintain secure connections.
Implementing Helmet.js for Additional Security
What is Helmet.js?
Helmet.js is a middleware for Express applications that sets various HTTP headers to protect against common web vulnerabilities.
Installation
npm install helmet
Basic Usage
const express = require("express"); const helmet = require("helmet"); const app = express(); app.use(helmet()); app.get("/", (req, res) => { res.send("Hello, secure world!"); }); app.listen(3000, () => { console.log("Server is running on port 3000"); });
Configuring Helmet.js
Customize security headers, such as Content Security Policy (CSP) and Referrer Policy, to enhance application security.
Utilizing Social Sign-In and API Keys
Social Sign-In
Social sign-in simplifies the login process by allowing users to authenticate using existing social network accounts, reducing friction and enhancing user experience.
API Keys
API keys serve to identify and authorize projects making API calls, ensuring secure interactions with external services.
Understanding OAuth and Its Implementation
OAuth (Open Authorization) is a widely adopted standard for access delegation that allows users to grant third-party applications limited access to their information without sharing passwords. This mechanism not only enhances security but also improves user experience by enabling applications to interact seamlessly on behalf of users.
The OAuth 2.0 Authorization Code Flow
One of the most common OAuth flows is the OAuth 2.0 Authorization Code Flow, which consists of several key steps:
- User Authorization: The user is redirected to an authorization server, where they log in and grant permission to the application.
- Authorization Code: Once the user consents, the authorization server redirects back to the application with an authorization code.
- Token Exchange: The application uses this authorization code to request an access token from the authorization server.
- Access Token: This access token allows the application to access protected resources on behalf of the user.
OAuth in Action: Single Sign-On (SSO)
Single Sign-On (SSO) is another powerful application of OAuth, allowing users to log in once and gain access to multiple applications without needing to re-enter their credentials. Here’s how it typically works:
- Central Authentication: An authorization server (such as Keycloak or Okta) manages user authentication.
- Token Issuance: After successful authentication, the server issues tokens (ID token, access token).
- Token Usage: These tokens facilitate authentication and authorization across different applications, providing a seamless user experience.
Example: Implementing OAuth 2.0 in Node.js
Step 1: Redirect User to Authorization Server
When a user wants to log in, your application will redirect them to the authorization server:
app.get("/login", (req, res) => { const authorizationUrl = "https://authorization-server.com/auth"; const clientId = "your-client-id"; const redirectUri = "https://your-app.com/callback"; const scope = "openid profile email"; const state = "random-state-string"; res.redirect( `${authorizationUrl}?response_type=code&client_id=${clientId}&redirect_uri=${redirectUri}&scope=${scope}&state=${state}` ); });
Step 2: Handle Callback and Exchange Code for Token
Once the user has authorized, they will be redirected back to your application, where you will handle the callback:
app.get("/callback", async (req, res) => { const authorizationCode = req.query.code; const tokenUrl = "https://authorization-server.com/token"; const clientId = "your-client-id"; const clientSecret = "your-client-secret"; const redirectUri = "https://your-app.com/callback"; const response = await axios.post(tokenUrl, { code: authorizationCode, client_id: clientId, client_secret: clientSecret, redirect_uri: redirectUri, grant_type: "authorization_code", }); const accessToken = response.data.access_token; res.send(`Access Token: ${accessToken}`); });
Setting Up Google OAuth 2.0 Authentication with Passport.js
Step 1: Registering with Google
- **Create a Project
** in the Google Cloud Console. 2. Enable APIs & Services needed for your application. 3. **Create OAuth 2.0 Credentials** with the necessary details and authorized redirect URIs.
Step 2: Setting Up Middleware and Passport.js
Install the required packages:
npm install express passport passport-google-oauth20
Next, configure Passport.js:
const express = require("express"); const passport = require("passport"); const GoogleStrategy = require("passport-google-oauth20").Strategy; passport.use( new GoogleStrategy( { clientID: "YOUR_GOOGLE_CLIENT_ID", clientSecret: "YOUR_GOOGLE_CLIENT_SECRET", callbackURL: "http://www.example.com/auth/google/callback", }, function (accessToken, refreshToken, profile, done) { // Handle user creation or retrieval here } ) ); passport.serializeUser((user, done) => { done(null, user); }); passport.deserializeUser((obj, done) => { done(null, obj); });
Step 3: Set Up Express Middleware
Create your Express application and configure sessions:
const app = express(); app.use( require("express-session")({ secret: "your secret", resave: true, saveUninitialized: true, }) ); app.use(passport.initialize()); app.use(passport.session()); app.get( "/auth/google", passport.authenticate("google", { scope: ["profile"] }) ); app.get( "/auth/google/callback", passport.authenticate("google", { failureRedirect: "/" }), (req, res) => { res.redirect("/"); } ); app.listen(3000, () => { console.log("Server started on http://localhost:3000"); });
Session Management: Cookie-Based Authentication
1. Cookie-Based Authentication
This method involves storing a session identifier in a cookie on the client’s browser, allowing for user authentication on subsequent requests.
2. Server vs. Client-Side Sessions with Cookies
- Server-Side Sessions (Stateful Cookies): The server maintains session data, making it more secure but requiring resources to manage sessions.
- Client-Side Sessions (Stateless Cookies): All necessary information is stored in the cookie itself, which can reduce server load but poses security risks if not managed correctly.
3. Session Middleware in Express
To manage sessions in an Express application, you can use the
express-session
middleware:
const express = require("express"); const session = require("express-session"); const app = express(); app.use( session({ secret: "your secret key", resave: false, saveUninitialized: true, cookie: { secure: false }, // Set to true if using HTTPS }) ); app.get("/", (req, res) => { if (req.session.views) { req.session.views++; res.send(`Number of views: ${req.session.views}`); } else { req.session.views = 1; res.send("Welcome to the session demo. Refresh!"); } }); app.listen(3000, () => { console.log("Server started on http://localhost:3000"); });
Protecting Routes with Authentication
To restrict access to certain endpoints using Passport.js, define middleware that checks if a user is authenticated:
function ensureAuthenticated(req, res, next) { if (req.isAuthenticated()) { return next(); } res.redirect("/login"); // Redirect to login page if not authenticated } // Apply the middleware to protect routes app.get("/dashboard", ensureAuthenticated, (req, res) => { res.send("Welcome to your dashboard, " + req.user.username); });
Implementing Logout Functionality
Implementing logout in your application is straightforward. You simply need to call
req.logout()
:
app.get("/logout", (req, res) => { req.logout((err) => { if (err) { return next(err); } res.redirect("/"); // Redirect to home page after logout }); });
Conclusion
By implementing robust authentication and authorization mechanisms, utilizing SSL/TLS for secure communications, and adopting OAuth for seamless third-party integration, you can significantly enhance the security of your Node.js applications. Following best practices not only protects user data but also ensures a reliable and trustworthy application environment. Embrace these strategies to provide your users with the secure and convenient access they expect in today's digital age.