Mastering JavaScript
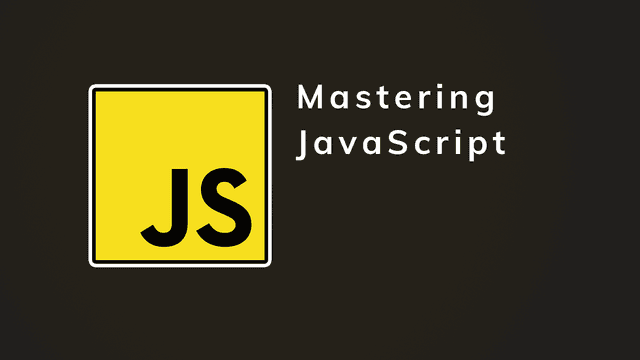
JavaScript powers the web - it's the most important programming language you need to know as a web developer.
Comprehensive Guide to JavaScript
Introduction
JavaScript is an essential programming language that powers the web. Originally developed to enhance web pages, it has evolved into a versatile tool used for both front-end and back-end development. Its foundation lies in the ECMAScript standard, enabling it to support multiple programming paradigms, including event-driven, functional, and object-oriented programming. This guide delves into the core concepts, features, and best practices of JavaScript to equip you with a deeper understanding of this powerful language.
JavaScript Engine, Interpreter, Compiler, and JIT Compiler
JavaScript Engine
A JavaScript engine is a software component responsible for executing JavaScript code. Different browsers utilize different engines. For example:
- V8: Developed by Google, used in Chrome and Node.js.
- SpiderMonkey: Mozilla’s engine used in Firefox.
- JavaScriptCore: Also known as Nitro, used in Safari.
Interpreter vs. Compiler vs. JIT Compiler
-
Interpreter: Executes code line-by-line, translating it into machine code at runtime. This allows for immediate execution but can lead to slower performance.
-
Compiler: Translates the entire code into machine code before execution. This generally improves performance as the machine code is directly executed.
-
JIT Compiler (Just-In-Time Compiler): Combines both approaches by compiling portions of code during execution, optimizing performance dynamically based on usage patterns.
Garbage Collection and Hoisting
Garbage Collection
JavaScript employs automatic memory management known as garbage collection. This process identifies and reclaims memory occupied by objects that are no longer in use, helping prevent memory leaks and optimize resource usage.
Hoisting
Hoisting is a fundamental concept where variable and function declarations are moved to the top of their respective scopes during compilation. This means that you can reference variables and functions before their actual declaration in the code. However, only declarations are hoisted, not initializations.
ECMAScript
ECMAScript is the standardized specification that serves as the foundation for JavaScript. It is essential to stay updated on ECMAScript versions, with ES6 (ECMAScript 2015) introducing numerous significant features that enhance the language's capabilities.
JavaScript Types and Variables
Types
JavaScript has several data types, categorized into primitive and non-primitive types:
- Primitive Types: Number, String, Boolean, Null, Undefined, Symbol, BigInt.
- Objects: Complex data structures that can hold collections of values and more complex entities.
Variables
Variables in JavaScript can be declared using:
- var: Function-scoped and can be redeclared.
- let: Block-scoped, allowing for more controlled variable scope.
- const: Also block-scoped, but cannot be reassigned after declaration.
Conditions and Logical Operators
Conditions
JavaScript provides several constructs for conditional logic, including:
- if, else if, else: Standard control flow statements.
- switch: A control statement that executes different parts of code based on the value of an expression.
Logical Operators
Logical operators are used to combine or invert boolean expressions:
- && (AND): Returns true if both operands are true.
- || (OR): Returns true if at least one operand is true.
- ! (NOT): Inverts the truth value of the operand.
Functions
Functions are fundamental building blocks in JavaScript. They can be defined in several ways:
- Function Declarations: Defined using the
keyword.function
- Arrow Functions: A more concise syntax introduced in ES6, allowing for lexical scoping of
.this
- Methods: Functions defined within objects.
Data Structures
Arrays
Arrays are ordered collections of values that can hold elements of any type, including other arrays.
Objects
Objects are collections of key-value pairs, serving as the primary data structure in JavaScript.
Maps and Sets
Introduced in ES6, Maps are key-value pair collections, while Sets hold unique values, making them useful for eliminating duplicates.
Looping
JavaScript provides several looping constructs:
- for: Traditional loop for iterating with a counter.
- while: Executes as long as the condition is true.
- do...while: Similar to while, but guarantees at least one execution.
- for...of: Introduced in ES6 for iterating over iterable objects like arrays and strings.
ES6 vs. ES5
ES6
Introduced numerous features to improve code readability and functionality, such as:
andlet
for better variable management.const
- Arrow functions for concise syntax.
- Classes for a clearer syntax for creating objects.
- Template literals for easier string interpolation.
- Destructuring for extracting values from arrays or objects.
ES5
The earlier version of JavaScript, lacking many of the modern features that enhance the developer experience.
Advanced Functions
Closures
Closures allow functions to retain access to their lexical scope, even when executed outside that scope.
Currying
Currying transforms a function that takes multiple arguments into a series of functions, each taking a single argument, allowing for more flexible function invocation.
Compose
Composing functions means creating a new function by combining multiple functions, enhancing code reusability.
Avoiding Side Effects and Functional Purity
Writing functions that do not alter external state and always return the same output for the same input is key to functional programming principles.
Destructuring
Destructuring is a powerful feature that allows for unpacking values from arrays or properties from objects into distinct variables, simplifying code and enhancing readability.
Advanced Objects
Reference Type
In JavaScript, objects are reference types. This means that when you assign an object to a variable, you are assigning a reference to that object, not a copy. Thus, multiple variables can point to the same object in memory.
Context and this
this
The value of
this
in JavaScript is determined by the context in which a function is called. Understanding this
is crucial for managing object-oriented code effectively.
Instantiation
Instances of objects can be created using constructors, allowing for the creation of multiple objects with similar properties and methods.
IIFE (Immediately Invoked Function Expressions)
IIFEs are functions that execute immediately after creation, often used to create a private scope and avoid polluting the global namespace.
call(), apply(), bind()
These methods allow developers to control the value of
this
within functions, enabling more flexible function invocation and context manipulation.
Pillars in JavaScript
Closures
Closures enable powerful programming techniques by capturing variables from their surrounding scope.
Prototypal Inheritance
JavaScript's inheritance model is based on prototypes, allowing objects to inherit properties and methods from other objects.
Functions as First-Class Citizens
Functions can be treated as first-class citizens in JavaScript, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
Higher-Order Functions
Higher-order functions are those that take other functions as arguments or return them, enabling a functional programming style.
OOP vs. Functional Programming in JavaScript
OOP (Object-Oriented Programming)
JavaScript supports OOP principles, using objects and classes to encapsulate data and behavior. Key concepts include:
this
new
- Prototype-based inheritance
- ES6 classes
Functional Programming
Functional programming emphasizes immutability and pure functions, focusing on the transformation of data rather than changes to state. Key concepts include:
- Currying
- Partial application
- Pure functions
- Referential transparency
- Compose and pipe functions
Asynchronous JavaScript
Web APIs
JavaScript can interact with browser-provided APIs for asynchronous operations, enabling tasks like fetching data from a server without blocking the main thread.
Async/Await
Async/await syntax allows developers to write asynchronous code that looks and behaves like synchronous code, greatly improving readability.
Callbacks
Callbacks are functions passed as arguments to other functions, executed later, often used for asynchronous operations.
Microtask Queue and Task Queue
JavaScript uses a microtask queue for promise resolutions and a task queue for other asynchronous operations, enabling efficient management of execution order.
Promises
Promises represent the eventual completion or failure of an asynchronous operation, providing a cleaner alternative to callbacks.
Event Loop
The event loop is a core mechanism in JavaScript that manages the execution of asynchronous code, ensuring smooth and non-blocking operations.
Modules in JavaScript
Native ES Modules
Introduced in ES6, the ES module system allows developers to structure their code into reusable modules, promoting better organization and encapsulation.
CommonJS
Used in Node.js, CommonJS is a module system that employs
require
for importing and module.exports
for exporting.
UMD (Universal Module Definition)
UMD is a module format that works in both browsers and Node.js, providing compatibility across environments.
AMD (Asynchronous Module Definition)
AMD is a module definition standard that works in browsers, enabling asynchronous loading of modules.
IIFE for Module Creation
IIFEs are also used to create modules, encapsulating functionality and avoiding global namespace pollution.
Error Handling in JavaScript
Effective error handling is crucial for robust applications. JavaScript provides
try
, catch
, and finally
blocks to manage exceptions gracefully, allowing developers to respond to errors without crashing the application.
Conclusion
JavaScript is a dynamic and evolving language that underpins modern web development. From its core concepts of types and functions to advanced features like asynchronous programming and modularity, understanding JavaScript is essential for any developer. By mastering these principles, you will be well-equipped to tackle a wide range of programming challenges, whether you are building interactive web applications, server-side solutions, or anything in between.